Write a Python program to enter the the number of family members and t...
**Python program to enter the number of family members and their age and store this information in dictionary**
To write a Python program to enter the number of family members and their age and store this information in a dictionary, we can follow the below steps:
1. Take input from the user for the number of family members.
2. Create an empty dictionary to store the family members' information.
3. Use a for loop to iterate over the range of number of family members entered by the user.
4. For each iteration, take input from the user for the family member's name and age.
5. Store the family member's information in the dictionary with their name as the key and age as the value.
Here is the complete Python program that implements the above steps:
```python
# take input from the user for the number of family members
num_members = int(input("Enter the number of family members: "))
# create an empty dictionary to store the family members' information
family = {}
# use a for loop to iterate over the range of number of family members
for i in range(num_members):
# take input from the user for the family member's name and age
name = input("Enter family member's name: ")
age = int(input("Enter family member's age: "))
# store the family member's information in the dictionary
family[name] = age
# print the dictionary containing the family members' information
print(family)
```
**Explanation:**
Let's go through the program step by step to understand how it works.
1. We start by taking input from the user for the number of family members using the `input()` function. We convert the input to an integer using the `int()` function and store it in the variable `num_members`.
```python
num_members = int(input("Enter the number of family members: "))
```
2. Next, we create an empty dictionary called `family` to store the family members' information.
```python
family = {}
```
3. We use a for loop to iterate over the range of number of family members entered by the user. The range function generates a sequence of numbers from 0 to `num_members - 1`.
```python
for i in range(num_members):
```
4. For each iteration of the for loop, we take input from the user for the family member's name and age using the `input()` function. We store the name in the variable `name` and the age as an integer in the variable `age`.
```python
name = input("Enter family member's name: ")
age = int(input("Enter family member's age: "))
```
5. Finally, we store the family member's information in the dictionary `family` using the family member's name as the key and their age as the value.
```python
family[name] = age
```
6. After all the family members' information has been entered and stored in the dictionary, we print the dictionary using the `print()` function.
```python
print(family)
```
This will output the dictionary containing the family members' information.
Write a Python program to enter the the number of family members and t...
#Program to create a dictionary which stores name of family members and their age
#entering the number of family members from user
num=int(input("Enter the the number of family members:"))
count=1
family=dict() #Creating an empty dictionary
while count<=num:
name=input("Enter the name of family member:")
age=int(input("Enter age:"))
family[name]=age
count+=1
print("\n\nfamily_member\tage")
for k in family:
print(k,'\t\t',family[k])
Output:
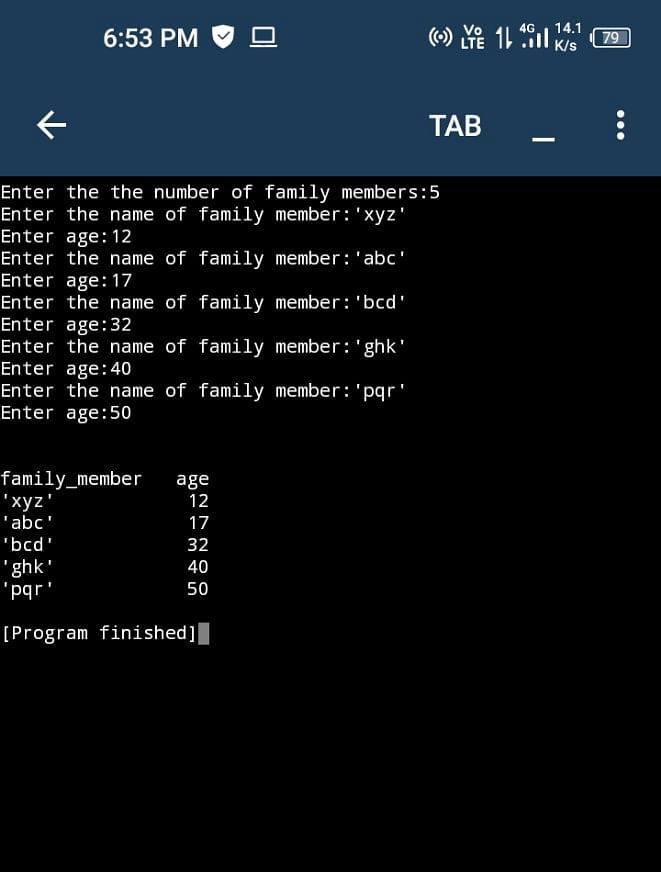
To make sure you are not studying endlessly, EduRev has designed Class 10 study material, with Structured Courses, Videos, & Test Series. Plus get personalized analysis, doubt solving and improvement plans to achieve a great score in Class 10.